Introduction to Programming in JavaScript
In this section you will discover some programming principles and concepts, so that you can start programming in the next chapter. If you have already coded using JavaScript (or a similar language), you can skip this chapter.
After completing this chapter, you will be able to:
- Understand basic programming concepts.
- Become comfortable with variables and functions.
- Understand conditional statements and Boolean logic.
- Understand some of the best practices for coding.
What is JavaScript
JavaScript is a scripting language that makes it possible to add interaction to web pages. JavaScript is usually embedded in a web page and executed within the browser; this why it is called a client-side scripting language, as it is executed in the browser (the client) rather than on a server.
JavaScript is often referred as ECMAScript; this is because while JavaScript was invented by Brendan Eich as a scripting language, ECMAScript is a standard for scripting languages, and JavaScript implements some of the features and specifications defined by ECMAScript.
JavaScript has the ability to access and amend the content of a web page; for example, with JavaScript, you can check the content of text fields, and you can display alert boxes and messages in the console window.
This being said, JavaScript also makes it possible to communicate with a server through technologies often referred as AJAX, whereby information is sent asynchronously to the server, and then processed accordingly.
JavaScript is usually embedded in an HTML file so that it can be executed by the browser; so in order to code your first JavaScript programme, you may need to have some understanding of HTML.
HTML and JavaScript
Before we start with JavaScript, let’s look at the structure of an HTML file to get to understand how JavaScript and HTML work together.
When you open a page on the internet, it is probably an HTML page. HTML stands for Hyper Text Markup Language and is a language that is used to define the content of a page. It consists of nested nodes organized hierarchically. After reading the HTML code for a page, the browser will in turn display its content as specified in the HTML file.
An HTML document usually includes two key parts call the head and the body, and that can typically be structured as described in the next code snippet:
<!DOCTYPE html> <html> <head> <title>My first html page</title> </head> <body> Hello world<br> Click here for google. </body> </html>
In the previous code:
- We declare the doctype, in our case, we will be using HTML5, and the corresponding doctype is HTML.
- We open the tag called <HTML> which corresponds to the entire document.
- We then include a nested tag called <HEAD> that will include information that will not be displayed on the page; for example, the title of the page, JavaScript code, or styling preferences.
- We then close the HEAD portion of the document using the tag </HEAD>
- We open the tag for the body <BODY> and add content that will be displayed on the page.
- We then close the body of the document using the tag </BODY>.
- We finally close the document using the tag </HTML>.
HTML files are usually saved with the extension .html, or .htm.
From the code above, we can infer a few rules and good practices for HTML documents:
- Each opening tag has a corresponding closing tag (e.g. <HTML> and </HTML>)
- It is good practice to use tabulation to keep the code neat and easily readable. Corresponding opening and closing tags are usually tabulated at the same level.
- Each pair of corresponding tags is nested within the “parent” tag (e.g. BODY tags are both inside the HTML tags).
- The <HTML> section is the topmost container of an HTML document
- <HEAD>: This section will contain information about the style of the document, the scripts in this page and the title of the page. In general, it contains information that is not considered to be document content (i.e., information that is not meant to be displayed).
- <TITLE>: These tags identify the content of the document.
- <BODY>: These tags contain the content of the document (what will be visible).
- <BR>: This tag includes a line break.
In the next sections, you will start to write your own JavaScript code. For this purpose, you will need to use a code editor.
Now, there are many code editors that you can use for this purpose, from very simple to sophisticated.
While simple text editors such as notepad are sufficient for JavaScript, more advanced code editors such as Sublime Text (my favorite), Atom, Brackets, or Vim might give you a significant advantage because they include, amongst other things, the following features that may speed-up the coding process:
- Text highlighting: so that you code is clearer to read and to modify.
- Auto-completion: so that you don’t have to remember the name of all the variables in your code, or the name of some common functions.
- Error highlighting: so that some obvious errors are highlighted before you even test your code.
You can download some common and free editors using the following links:
- Sublime Text: https://www.sublimetext.com/
- Atom: https://atom.io/
- Brackets: http://brackets.io/
- Vim: https://www.vim.org/
Browser Compatibility
JavaScript can be used in most current browsers, although some don’t implement all its features. The code presented in this book was written and tested in FireFox 60 for Mac OS and it should work in your own browser. This being said, alternative code is provided in case of possible incompatibilities with some browsers.
Creating your first page
After this brief introduction to HTML, it is now your turn to code.
So that you get to experiment with your first HTML page, please do the following:
- Open a text editor of your choice. If you are using Sublime Text, select File | New File from the top menu.
- Type the following code in the new document:
<!DOCTYPE html> <html> <head> <title>My first html page</title> </head> <body> Hello world<br> Next line. </body> </html>
In the previous code:
- We declare our document as HTML5 thanks to the doctype.
- We then add a title to our document using the tag <TITLE> that is nested within the <HEAD> tag.
- After closing the <HEAD> tag, we then open the <BODY> tag and add some text that will be displayed onscreen.
- The text Hello World is followed by the tag <BR> which corresponds to a line break.
- This line break is then followed by the text Next Line.
Note that each nested tag has been indented using a tabulation so that corresponding opening and closing tags appear at the same level and to the right of its parent. This is a good practice for clear and easily understandable code. It is also a very good way to check that all your tags are properly closed.
Once you have typed these lines, we can then save the file:
- Save this file as myFirstFile.html in a location of your choice. If you are using Sublime Text, please select File | Save As from the top menu.
When you save your HTML file, please ensure that use the extension .html, otherwise your computer will not know that this file is to be opened by a browser.
- Once the code has been saved, please open this file with your browser: navigate to the folder where you have saved the HTML file and double click on it, this should open the page in your default browser. Alternatively, you can left-click on the file and select the application that you’d like to use to open the file from the contextual menu.
- Once this is done, you should see a page in your browser with the title “My First HTML Page”, along with some text in the body of the document, as illustrated in the next figure.
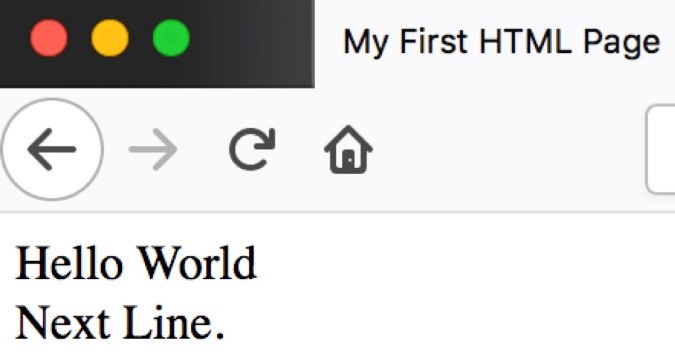
Figure 1‑1: Opening your first HTML page
Now that we have a basic understanding of the structure of an HTML document, we can start to see how JavaScript can be added to this page.
Typically, JavaScript code is added to an HTML file using the <SCRIPT> tags. These tags can be added to the HEAD or BODY of the document.
- Please open the document myFirstScript.html that you have just created in the text editor of your choice.
- Add the new code (highlighted in bold) to this file.
<!doctype html> <html> <head> <title>My first html page</title> <script> alert("hello from the head"); </script> </head> <body> Hello world<br> <script> alert("Hello from the body"); </script> </body> </html>
In the previous code:
- We add a script tag in the HEAD of our document. The code within these tags displays an alert box.
- The code is followed by a semicolon.
- We also add a <SCRIPT> tag in the BODY of our document. The code within these tags displays an alert box.
Note that is some special circumstances, it may be preferable to use a script in the BODY of the document rather than the HEAD so that allHTML elements have been read and displayed by the browser before JavaScript code is executed.
- In both cases, the script will be executed by the browser.
Also note that the previous code assume that you are enabling pop-up windows in your browser. In Internet explorer, this can be done using the menu Tools | Options, and in Mozilla Firefox, you just need to select the “hamburger” menu in the top-right corner, and then select Options.
Once you have updated the script, please save your changes (CTRL + S) and refresh the corresponding browser page (CTRL + R or APPLE+R) where your page is open (or open your page in a browser of your choice). You should see that the message “Hello from the head” appears. Then, as soon as you press the “OK” button, the message “Hello from the Body” should appear.
Well done, you have written your first piece of JavaScript code!
While this code consisted of very simple instructions to display a message onscreen, it shows how you can add some JavaScript code into your web pages.
So, now that we have written our first script, the next sections will look at how JavaScript programs are structured.
Introduction to JavaScript Syntax
With JavaScript, you are communicating with the browser asking it to perform actions and using a language or a set of words bound by a syntax that the browser and you know. This language consists of keywords, key phrases, and a syntax that ensures that the instructions are understood properly. In computer science, these instructions need to be exact, precise, unambiguous, and with a correct syntax. In other words, it needs to be exact.
A syntax consists of a set of rules to be followed when writing your code. In the next section, we will learn how to use the proper syntax for JavaScript by combining the following:
- Statements.
- Comments.
- Variables.
- Operators.
- Assignments.
- Data types.
- Functions.
- Objects.
- Events.
- Comparisons.
- Type conversions.
- Reserved words.
- Messages to the console windows.
- Declarations.
- Calls to functions.
The list may look a bit intimidating but, not to worry, we will explore these in the next sections, and you will get to know and use them smoothly.
statements
When you write a piece of code, as we have done in the HTML files that you have just created, you need to tell the system to execute your instructions (e.g., to print information) using statements. A statement is literally an order or something that you ask the system to do. For example, in the next line of code, the statement will tell the browser to display a message using an alert window:
alert("Hello Word");
When writing statements, there are a few rules that you need to know:
- Order of statements: in a script, each statement is executed sequentially in order of appearance. For example, in the next example, the code will display hello, then word; this is because the associated statements appear in that order in the script.
alert("hello"); alert("world");
- Statements are separated by semi-colons (i.e., semi-colon at the end of each statement). This is not required all the time (e.g., end of a line), but it is good practice to follow this rule for consistency and clarity.
Note that several statements can be added on the same line, as long as they are separated by a semi-colon.
- For example, the next line of code has a correct syntax.
alert("hello");alert ("world");
- Multiple spaces are ignored for statements; however, it is good practice to add spaces around the operators such as +, -, /, or % for clarity. For example, in the next example, we state that a is equal to b. There is a space both before and after the operator =.
a = b;
- Statements to be executed together (e.g., based on the same condition) can be grouped using what is usually referred to as code blocks. In JavaScript, code blocks are symbolized by curly brackets (e.g., { or }). So, in other words, if you needed to group several statements, we would include them all within the same curly brackets, as follows:
{ alert ("hello stranger!"); alert ("today, we will learn about scripting"); }
As we have seen earlier, a statement usually employs or starts with a keyword (i.e., a word that the computer knows). Each of these keywords has a specific purpose and the most common ones (at this stage) are used for:
- Displaying an alert box: the keyword is alert.
- Printing a message in the console window: the keyword is console.log.
- Declaring a variable: the keyword is var.
- Declaring a function: the keyword is function.
- Marking a block of instructions to be executed based on a condition: the keywords are if and else.
- Exiting a function: the keyword is return.
Now that you know a bit more about statements, let’s modify your initial scripts to include more statements:
- Please open the scrip myFirstScript.html in the editor of your choice.
- Add the following code:
<SCRIPT> alert("Hello from the head"); { alert ("this is my first script"); alert ("This statements and the previous one belong to the same block of instructions"); } </SCRIPT>
In the previous code:
- We have created a block of instructions that starts with an opening curly bracket and that ends with a closing curly bracket.
- Within this block, we have created two statements based on alert boxes; both statements are ending with a semi-colon.
You can now save your code and refresh the corresponding browser page; you should see two additional messages displayed on the screen (i.e., the ones that you have just added).
For now, using blocks of instructions may look like it will not make much of a difference to your coding, and this is true for now. This being said, blocks of instructions are very helpful, as we will see later, especially when we need to tell the browser to execute a list of instructions. This is because, in this case, instead of telling the browser to execute each instruction individually, we can instead, ask for a block of instructions to be executed.
Comments
In JavaScript, you can use comments to explain the code and to make it more readable. This is important for you, as the size of your code increases, and it is also important if you work as part of a team, so that team members can understand your code and make amendments in the right places if and where necessary.
When code is commented, it is not executed and there are two ways to comment your code in JavaScript using single or multi-line comments.
In single-line comments, a double forward slash is added at the start of a line or after a statement, so that this line (or part thereof) is commented, as illustrated in the next code snippet.
//the next line displays hello onscreen alert ("hello");
In multi-line comments, any text between /* and */ will be commented (and not executed). This is also refereed as comment blocks.
/* the next lines after the comments displays the message hello onscreen we then display another message */ alert("Hello"); alert("Another message");
In addition to providing explanations about your code, you can also use comments to prevent part of your code to be executed. This is very useful when you would like to debug your code and find where the error or bug might be, using a very simple method. By commenting sections of your code, and by using a process of elimination, you can usually find the issue quickly. For example, you can comment all the code and run the script; then comment half the code, and run the script. If it works without no errors, it means that the error is within the code that has been commented, and if it does not work, it means that the error is in the code that has not been commented. In the first case (when the code works), we could then just comment half of the portion of the code that has already been commented. So, by successively commenting more specific areas of our code, we can get to discover what part of the code includes the bug. This process is often called dichotomy (as we successively divide a code section into two).
Let’s use comments in our script:
- Please open the script myFirstScript.html in the editor of your choice.
- Modify the code as follows (new code in bold).
<!doctype html> <html> <head> <title>My first html page</title> <script> //alert("hello from the head"); { alert ("This is my first script"); alert ("This statements and the previous one belong to the same block of instructions"); } </script> </head> <body> Hello world<br> Click here for google. <script> //alert("hello from the body"); </script> </body> </html>
In the previous code, we comment the message alert(“Hello from the head”) andthe message alert(“Hello from the body”).
Now that you have made these changes, please save your code and refresh the corresponding page in your browser; you should see that, this time, only two messages are displayed on screen, because the two other messages (or statements) have been commented.
We could also use multi-line comments as follows.
<script> //alert("Hello from the head"); /* { alert ("this is my first script"); alert ("This statements and the previous one belong to the same block of instructions"); } */ </script>
In the previous code, we used a multi-line comment. This means that the two lines comprised between /* and */ , while they belong to the file, will not be executed.
If you save your code and refresh the corresponding web page, you should see that no message is displayed onscreen, because all the alert statements have been commented.
Variables
Now that you know a bit more about statements and comments, we will start to learn more about variables, which are quite important in JavaScript, as you will probably use them in most of your programs to perform calculations or to work with text.
So what is a variable?
A variable is a container. It includes a value that may change over time. When using variables, we usually need to: (1) declare the variable, (2) assign a value to this variable, and (3) possibly combine this variable with other variables using operators.
Let’s look at an example of how variables can be employed.
var myAge;//we declare the variable myAge = 20;// we set the variable to 20 myAge = myAge + 1; //we add 1 to the variable myAge
In the previous example, we declare a variable called myAge, we set it to 20 and we then add 1 to it.
Note that in the previous code, we have assigned the value myAge + 1 to the variable myAge. The = operator is an assignment operator; in other words, it is there to assign a value to a variable and is not to be understood in a strict algebraic sense (i.e., that the values or variables on both sides of the = sign are equal).
When using variables, there are a few things that we need to determine including their name, type and scope:
- Name of a variable: A variable is usually given a unique name so that it can be identified uniquely. The name of a variable is usually referred to as an identifier. When defining an identifier, it can, contain letters, digits, a minus, an underscore or a dollar sign, and it usually begins with a letter. Identifiers cannot be keywords (e.g., such as if).
- Type of variable: variables can hold several types of data including numbers (e.g., numbers with or without decimals), text (i.e., these are usually referred as strings), arrays, Boolean values (e.g., true or false), or objects (e.g., we will look at this concept later), as illustrated in the next code snippet.
var myName = "Patrick";//the text or string is declared using double quotes var currentYear = 2015;//the year needs no decimals and is declared as an integer var width = 100.45;//width is declared as a number with decimals
- Variable declaration: a variable needs to be declared before its and the declaration is performed using the keyword var. At the declaration stage, the variable does not have to be assigned a value, as this can be done later. The next code snippet illustrates a variable declaration.
var myName; myName = "My Name";
In the previous example, we declare a variable called myName and it is then assigned the value “My Name”.
- Scope of a variable: a variable can be accessed (i.e., referred to) in specific contexts that depend on where in the script the variable was declared. We will look at this concept later.
Common variable types include:
- Strings: same as text.
- Numbers without decimals: these are integers (e.g., 1, 2, 3, etc.).
- Numbers with decimals: for example, 1.2, 1.3, etc.
- Boolean variables: for example, true or false.
- Objects: these are structures that include several attributes (i.e., properties) and associated functions (i.e., methods).
- Arrays: a collection of variables of objects of the same type.
Note that it is possible to declare several variables in the same statement, as follows:
var name="Pat", location = "Ireland", year = 2018;
So let’s experiment with variables using our script:
- Please open the script myFirstScript.html.
- Add the following code to it (new code in bold)
<head> <title>My first html page</title> <script> var myname = "patrick"; alert ("Hello " + myname); //alert("hello from the head"); /* { alert ("this is my first script"); alert ("this statements and the previous one belong to the same block of instructions"); } */ </script> </head>
In the previous code:
- We declare a variable called myName.
- It becomes a string as we assign the word Patrick (surrounded by double quotes) to this variable.
- We then use the alert keyword to display a message that starts with “Hello” followed by the content of the variable myName.
Note that if we had omitted the quotes around the word “Patrick“, the browser would have assumed that we referred to a variable called Patrick as opposed to a string for which the content is “Patrick”. Using the latter would have caused an error. We will come back to this later and see how it is possible to locate an error in your scripts.
Operators
Once we have declared and assigned a value to a variable, we can use operators to modify or combine this variable with other variables. There are different types of operators available in JavaScript, including arithmetic operators, assignment operators, comparison operators and logical operators.
So let’s look at each of these.
- Arithmetic operators are used to perform arithmetic operations including additions, subtractions, multiplications, or divisions. Common arithmetic operators include +, -, *, /, or % (modulo) and their use is illustrated in the next code snippet.
var number1 = 1;// the variable number1 is declared var number2 = 1;// the variable number2 is declared var sum = number1 + number2;// adding two numbers and store them in sum var sub = number1 - number2;// subtracting two numbers and store them in sub
- Assignment operators can be used to assign a value to a variable and include =, +=, -=, *=, /= or %= and their use is illustrated in the next code snippet.
var number1 = 1;
var number2 = 1; number1+=1; //same as number1 = number1 + 1; number1-=1; //same as number1 = number1 - 1; number1*=1; //same as number1 = number1 * 1; number1/=1; //same as number1 = number1 / 1; number1%=1; //same as number1 = number1 % 1;
Note that the = operator, when used with strings, will concatenate them (i.e., add them one after the other to create a new string). When used with a number and a string, the same will apply and the result is a string (for example “Hello”+1 will result in “Hello1“).
- Comparison operators are often used for conditions to compare two values. Comparison operators include ==, !=, >, <, >= and >= and their use is illustrated in the next code snippet.
if (number1 == number2); //if number1 equals number2 if (number1 != number2); //if number1 and number2 have different values if (number1 > number2); //if number1 is greater than number2 if (number1 >= number2); //if number1 is greater than or equal to number2 if (number1 < number2); //if number1 is less than number2 if (number1 <= number2); //if number1 is less than or equal to number2
Let’s use some of these operators in your own code:
- Please open the script myFirstScript.html.
- Add the following code to it).
<script> var myname = "patrick"; alert ("Hello " + myname); var yearofbirth = 1990; var age = 2018-yearofbirth; alert ("you are " + age + " years old");
In the previous code:
- We declare the variables yearOfBirth and age.
- We subtract the year of birth from the current year.
- The result of this subtraction is saved in the variable called age.
- We display the result.
Conditional statements
While we have looked at simple variables such as numbers or text, and how to modify them through operators, we will now look at conditional statements.
Conditional statements are statements that are performed based on a condition, hence their name. Their syntax is usually as follows:
if (condition) statement;
This means if the condition is verified (or true) then (and only then) the statement is executed. When we assess a condition, we test whether a declaration (or statement) is true.
For example, by typing if (a == b), we mean “if it is true that a equals b”. Similarly, if we type if (a>=b) we mean “if it is true that a equals or is greater than b”
As we will see later, we can also combine conditions. For example, we can decide to perform a statement if two (or more) conditions are true. For example, by typing if (a == b && c == 2) we mean “if a equals b and c equals 2”. In this case using the operator && means AND, and that both conditions will need to be true. We could compare this to making a decision on whether we will go sailing tomorrow.
For example, we could translate the sentence “if the weather is sunny and the wind speed is less than 5km/h then I will go sailing” as follows.
if (weatherIsSunny == true && windSpeed < 5) IGoSailing = true;
When creating conditions, as for most natural languages, we can use the operator OR noted ||. Taking the previous example, we could translate the following sentence “if the weather is too hot or the wind is faster than 5km/h then I will not go sailing ” as follows.
if (weatherIsTooHot == true || windSpeed >5) IGoSailing = false;
Another example could be as follows.
if (myName == "Patrick") alert("Hello Patrick"); else alert ("Hello Stranger");
In the previous code, we display the text “Hello Patrick” if the variable myName equals to “Patrick“; otherwise, we display the message “Hello Stranger“.
When we assess true and false conditions, we are effectively applying what is called Boolean logic. Boolean logic deals with Boolean numbers that have two values 1 and 0 (or true and false). By evaluating conditions, we are effectively processing Boolean numbers and applying Boolean logic. While you don’t need to know about Boolean logic in depth, some operators for Boolean logic are important, including the ! operator. It means NOT or “the opposite of“. This means that if a variable is true, its opposite will be false, and vice versa. For example, if we consider the variable weatherIsGood = true, the value of !weatherIsGood will be false (its opposite). So the condition if (weatherIdGood == false) could also be written if (!weatherIsGood) which would literally translate as “if the weather is NOT good”.
Let’s experiment with conditional statements:
- Please open the script myFirstScript.html.
- Add the following code (new code in bold) at the beginning of the script:
<title>my first html page</title> <script> var nameofuser = prompt("Please enter your name",""); if (nameofuser == "patrick") alert ("Hello patrick"); else alert ("Hello stranger");
In the previous code:
- We declare the variable nameOfUser.
- We then ask (or “prompt”) the user to enter his or her name using the prompt method, which is a method provided by JavaScript. The first parameter is the label of the box (i.e., “Please enter your name)” and the second parameter, which is an empty string at present, defines the text that will be displayed as the default answer. Once the name has been entered by the user, it is stored in the variable nameOfUser.
- We then execute a conditional statement based on the value of the variable nameOfUser. If the text entered is “Patrick” (note that this is case-sensitive, so the browser is looking for this exact string, accounting for the case of each letter within), then the message “Hello Patrick” is displayed. Otherwise, the message “Hello Stranger” is displayed.
You can now save your code and refresh the corresponding web browser page, and you should see the following box:
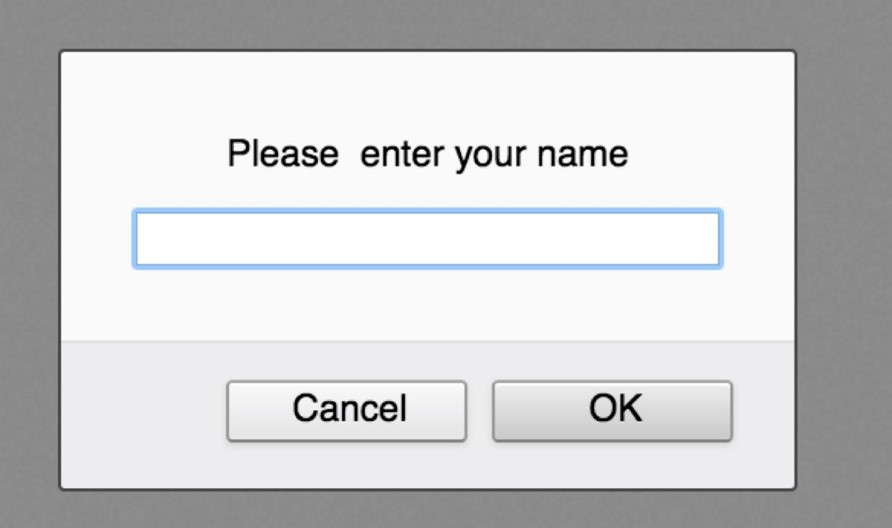
Figure 1‑2: Using the Prompt function
- As you enter the name “Patrick” and press the button labelled “OK“, the following message should appear.
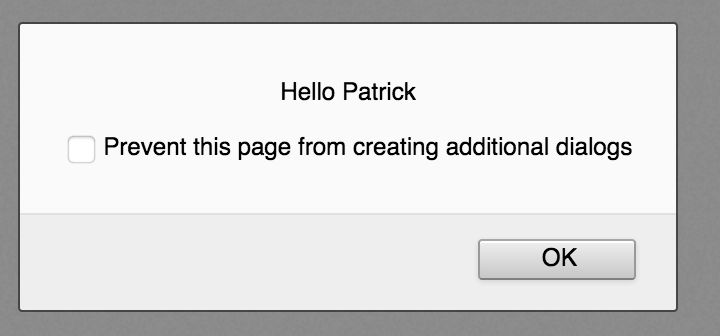
Figure 1‑3: Displaying a message based on the user input
- You can then click on the button labeled “OK“.
Now that we have used conditional statements for strings, we can also experiment with numbers. So, please amend the code in your you HTML file (new code in bold).
<title>My first html page</title> <script> /*var nameofuser = prompt("please enter your name",""); if (nameofuser == "patrick") alert ("hello patrick"); else alert ("hello stranger");*/ var result = prompt("What is 1 + 1",""); if (result == "2") alert ("Well done"); else alert ("Sorry, wrong answer");
In the previous code:
- We comment the previous code.
- We declare a variable called result.
- We then ask (or “prompt”) the user to enter his/her guess using the prompt method. The first parameter is the label of the box and the second parameter which is an empty string at present, defines the text that will be displayed as a default answer. Once the answer has been entered, it is stored in the variable result.
- We then perform a conditional statement based on the value of the variable result. If the text entered is “2“, then the message “Well done” is displayed, otherwise, the message “Sorry Wrong Answer” is displayed instead.
Note that in the previous example, we ask for a number to be entered; however, because it is entered in a prompt box, it will be considered as a string by JavaScript. This is the reason why we are using double quotes around the number “2” when testing the answer; ideally, and we will look into this later, we could have converted the entry to a number and the code could have looked like as follows:
var result = prompt("What is 1 + 1",""); resultAsNumber = parseInt(result); //if (result == "2") alert ("Well done"); if (resultAsNumber == 2) alert ("Well done"); else alert ("Sorry, wrong answer");
In the previous code:
- We declare the variable resultAsANumber.
- We convert the answer provided by the user, which is a string so far, to an integer (i.e., a number with no decimals).
- This new number is then stored in the variable called resultAsANumber.
- The conditional statement now uses the number 2 as a condition as opposed to the string “2“.
Note that if you want to copy/paste the code from this book, make sure that the double quotes are straight and not curled, otherwise, the browsers may perceive the curled quotes as an error.
You can now save your code and refresh the corresponding web browser page and you should see, as for the previous example, a prompt asking you to enter a number.
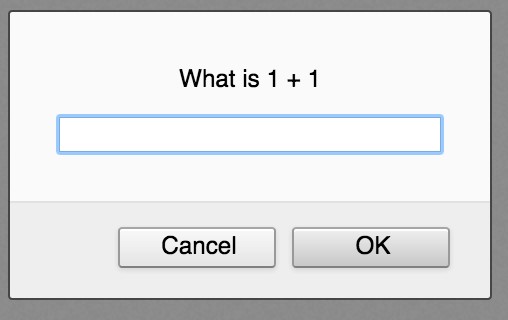
Figure 1‑4: Asking the user to enter a number
- After entering the number 2 and pressing the “OK” button, the following message should appear.
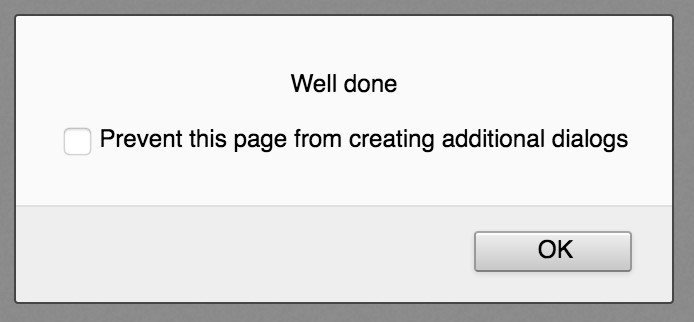
Figure 1‑5: Displaying feedback
Functions
Now that you can declare and combine numbers and strings, it is a good time to start looking into functions.
Functions can be compared to a friend or a colleague to whom you gently ask to perform a task, based on specific instructions, and to return information afterwards, if need be.
For example, you could ask your friend the following: “Can you please tell me when I will be celebrating my 20th birthday given that I was born in 2000”. So you give your friend (who is good at Math :-)) the information (i.e., the date of birth) and s/he will calculate the year of your 20th birthday and give this information back to you. So in other words, your friend will be given an input (i.e., the date of birth) and s/he will return an output (i.e., the year of your 20th birthday). Functions work exactly this way: they are given information (and sometimes not), perform an action, and then sometimes, if needed, return information.
In programming terms, a function is a block of instructions that performs a set of actions. It is executed when invoked (or put more simply called) from the script, or when an event occurs (e.g., when the player has clicked on a button; we will see more about events in the next section). As for variables, functions are declared and can also be called.
Functions are very useful because once the code for a function has been created, it can be called several times without the need to rewrite the same code all over again. Also, because functions can take parameters, a function can process these parameters and produce or return information accordingly; in other words, they can perform different actions and produce different outputs based on the input. As a result, functions can do one or all of the following:
- Perform an action.
- Return a result.
- Take parameters and process them.
A function is declared using the keyword function, as follows:
function nameOfTheFunction () { Perform actions here }
In the previous code the function does not take any input; neither does it return an output. It just performs actions.
function nameOfTheFunction (parameterName) { Perform actions here return value; }
In the previous code the function takes a parameter (i.e., an input), performs actions, and then returns a value. In this example, the parameter that is passed will be referred as parameterName within the function.
A function can be called using the () operator, as illustrated in the next code snippet:
nameOfTheFunction1(); nameOfTheFunction2(value); var test = nameOfFunction3(value);
In the previous code, a function is called with no parameter (first line), or with a parameter (second line). On the third line, a variable called test will be set with the value returned by the function nameOfFunction3.
Let’s experiment with functions and create our very own function:
- Please open your HTML file and comment your previous code as follows:
/*var result = prompt("What is 1 + 1",""); resultAsNumber = parseInt(result); //if (result == "2") alert ("Well done"); if (resultAsNumber == 2) alert ("Well done"); else alert ("Sorry, wrong answer"); */
- Then add the following code within the SCRIPT tags located in the head of the document:
<SCRIPT> var myAge = calculateAge (1990); alert ("My Age is " + myAge); function calculateAge(yearOfBirth) { return(2018-yearOfBirth); }
In the previous code:
- We declare a variable called myAge.
- We call the function calculateAge, passing the number 1990 as a parameter.
- The information (or value) returned by the function is stored in the variable myAge and then displayed using the alert function.
- The function calculateAge is declared; it takes one parameter called yearOfBirth. So any parameter passed to this function will be referred as yearOfBirth within this function.
- This function returns the result of the subtraction 2018 – yearOfBirth.
- When the function is called, yearOfBirth is equal to 1990; so it will return 28 (i.e., 2018-1990).
Please save your code, and refresh the corresponding web page, and you should see the following message.
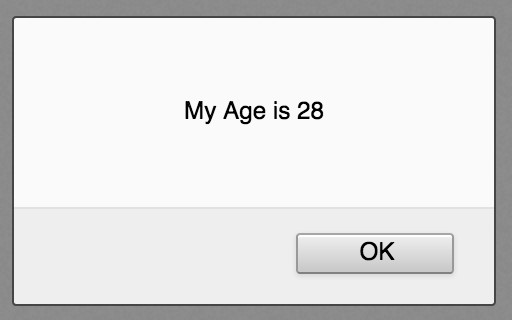
Figure 1‑6: Displaying the value returned by the function
Whenever you create variables in JavaScript, you will need to be aware of their scope so that you use it where its scope makes it possible for you to do so. This is particularly important if you use functions, as some variables will be used just locally, whereas others will be used throughout the script.
The scope of a variable refers to where you can use this variable in a script. In JavaScript, variables can be either global or local.
You can compare the terms local and global variables to a language that is either local or global. In the first case, the local language will only be used (i.e., spoken) by the locals, whereas the global language will be used (i.e., spoken) and understood by anyone whether they are locals or part of the global community.
- Global variables can be used anywhere in your script, hence the name global. These variables need to be declared at the start of the script (using the usual declaration syntax) and outside of any function. They can then be used anywhere in the script, as illustrated in the next code snippet.
var myVar; function function1() { myVar = 0; } function function2() { myVar = myVar + 1; }
In the previous code, the global variable myVar is declared at the start of the script; it is then initialized in function1, and updated in function2.
- Local variables are declared (and should only be used) within a specific function; hence the term local, because they can only be used locally, as illustrated in the next code snippet.
function function1() { var myVar:int; myVar = 0; } function function2() { var myVar2:int; myVar2 = 2; }
In the previous code, myVar is a local variable to the function function1, and can only be used within this function; myVar2 is a local variable to the function function2, and can only be used within this function.
If a variable is initialized in a method without being declared, it is considered as a global variable, as illustrated in the next code snippet.
function function3() { testGlobal = 3; } function3(); alert("value of test" + testGlobal);//this should display "value of test 3";
In the previous code, the variable testGlobal has not been defined using the var keyword, and it is therefore global and accessible throughout the script (i.e., inside and outside the function function3)
Note that it is always good to avoid what is called variable masking/shadowing, which occurs when you give a local variable the same name as a global variable in the same file, as this could lead to confusions.
So let’s experiment with variables and their scope:
- Please add the following code to the beginning of the SCRIPT area in the head of the document myFirstScript.html.
var myGlobalVariable = 3; myFunction(); alert ("The value of myLocalVariableFromMyFunction is"+myLocalVariableFromMyFunction); function myFunction() { alert ("The value of myGlobalVariable is"+myGlobalVariable); var myLocalVariableFromMyFunction = 4; }
In the previous code:
- We declare a global variable called myGlobalVariable; because it is declared outside any function, this variable will be global and accessible throughout our script.
- We then call the function myFunction.
- In the function called myFunction, we display the value of the variable myGlobalVariable and we also declare a local variable called myLocalVariableFromFunction and set it to 4.
- Finally, we try to display the value of the local variable myLocalVariableFromFunction from outside the function where it was first defined. Since this is a local variable, this should not be possible, and hence, this should trigger an error.
Please save your code and refresh the corresponding web browser page. You should see that the browser displays the value of the variable myGlobalVariable; however, nothing happens after this message. That is, the value of the global variable is not displayed onscreen. As you may have guessed, this is normal because we are trying to display the value of the local variable myLocalVariableFromFunction from outside the function where it was first defined. Since this is a local variable, this should not be possible, and hence, it should trigger an error.
In JavaScript, a script will be executed until an error occurs in this script, and this is exactly what has happened here; this being said, it would be great to see errors and possibly where they originate from, to be able to, if need be, detect and correct bugs in our code. Thankfully, errors are usually displayed in what is called the console window of your browser, and we will learn more about this window, in the next section.
Using the Console window
The console window is usually provided in most modern browsers and it makes it possible to display errors or messages that you can write from your code, essentially for debugging purposes. While in most browsers the console window can be accessed using the F12 key, it can also be accessed as follows:
- In Internet Explorer: Select Tools | Developer Tools | Console.
- In FireFox: Install the plugin called FireBug (http://getfirebug.com) and then select Tools | WebDevelpper | Web Console.
- In Safari: Install Firebug Lite (http://safari-extensions.apple.com) and then select Tools | WebDevelopper | Web Console.
Once you have activated the console window in your browser, it should appear at the bottom of the browser window, and look like the following figure:

Figure 1‑7: Using the console window
If your refresh the page that we have created so far (i.e., myFirstScrpit.html) and select the Console tab located at the bottom of the window, you should see the following messages in the console window.

Figure 1‑8: Reading error messages in the console window
This message means that the variable myLocalVariableFromFunction is not defined; this is because, as we have seen before, we are trying to access a variable outside its scope, that is, from outside the function myFunction where it was previously defined as a local variable.
You may also see, to the right of this message, some information about the location of the error, as illustrated in the next figure:

Figure 1‑9: Displaying the location of the error
In the previous figure, the console window specifies that the error was detected in the file called myFirstScript, at the line number 8 and row number 4. If you look at the HTML file, this corresponds exactly to the line where we have tried to access a local variable from outside its function and scope, as illustrated in the next figure.
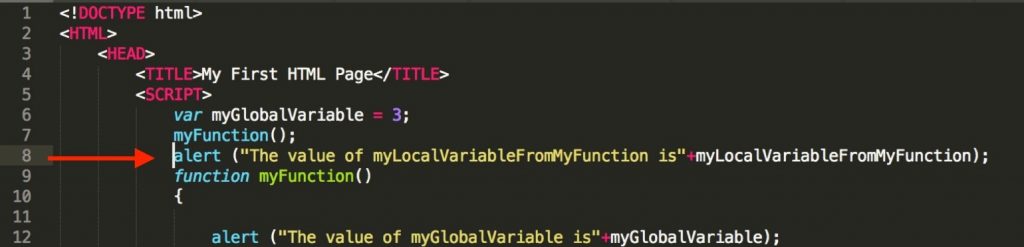
Figure 1‑10: Localizing errors and bugs
So as you can see, the console window is very useful to debug your code and to locate errors. This is very important because, when an error is detected, the browser will just stop to execute any further code, and it may be difficult to detect errors otherwise.
In addition to error messages, you can also display messages yourself in the console window, using the keyword console.log.
For example, the following code will display the sentence “Starting Function1” in the console window.
console.log (“Starting Function1”)
After this short introduction to the console window, let’s try to use this feature in our code:
- Please open the file myFirstScript.html.
- Modify the code as follows (new code in bold):
var myGlobalVariable = 3;
myFunction();
console.log(“Trying to access the local Variable”);
alert (“The value of myLocalVariableFromMyFunction is”+myLocalVariableFromMyFunction);
console.log(“Managed To Access the the local Variable “)
In the previous code we have added two messages to the console window. As you save and display the web page in your browser, you should see an alert box. After pressing the OK button from the alert box, you should also see one additional message in the console window, as illustrated in the next figure.

Figure 1‑11: Displaying messages in the Console window
As you can see, only the first console message was displayed (i.e., “Trying to access the local Variable”); this is because the next line creates an error; therefore, the next part of the code, including the second message, is not executed by the browser.
Storing all scripts in one file
Often, your JavaScript code will be stored in an HTML file; however, you can also use external JavaScript files to store all your scripts; this provides several advantages, including:
- All your scripts are stored in one place.
- You can avoid the duplication of your scripts and code, and hence save time coding.
- Your code is neater and easier to read and maintain.
So let’s say that you have created three functions that will be used throughout your pages, and that you would like these to be available in only one place, you could do the following:
- Create a file called, for example, myFunctions.js, with a content similar to the following:
function add() { } function test() { }
You could then use these in a different HTML file as follows:
<html> <head> <script src = "./myScript.js"></script> </head> </html>
In the previous code we specify that some of our code is stored in a file called myScript.js that is located in the same folder as the current HTML page.
A few things to remember when you create a script (checklist)
When you start coding, you will, as for any new activity, make small mistakes, learn what they are, improve your coding, and ultimately get better at writing your scripts. As I have seen in the past with students learning scripting, there are some common errors that are usually made; these don’t make you a bad programmer; on the contrary, it is part of the learning process because we all learn by trial and error, and making mistakes is part of the learning process.
So, as you create your first script, set any fear aside, try to experiment, be curious, and get to learn the language. It is like learning a new foreign language: when someone from a foreign country understands your first sentences, you feel so empowered! So, it will be the same with JavaScript, and to ease the learning process, I have included a few tips and things to keep in mind when writing your scripts, so that you progress even faster. You don’t need to know all of these by now (I will refer to these later on, in the next chapter), but it is so that you are aware of it and also use this list if any error occurs. So, watch out for these: 🙂
- Each opening bracket has a corresponding closing bracket.
- All variables are written consistently (e.g., spelling and case). The name of each variable is case-sensitive; this means that if you declare a variable myvariable but then refer to it as myVariable later on in the code, this may trigger an error, as the variable myVariable and myvariable, because they have a different case (upper-case V), are seen as two different variables.
- As much as possible, declare a variable prior to using it (e.g., var myVar).
- For each variable name, all words (except for the first word) have a capitalized initial. This is not a strict requirement, however, it will make your code more readable.
- For each function, all words (except for the first word) have a capitalized initial. This is not a strict requirement; however, it will make your code more readable.
- All statements are ended with a semi-colon. This is not a strict requirement; however, it will make your code more readable.
- For if statements the condition is within round brackets.
- For if statements the condition uses the syntax “==” rather than “=”.
- When calling a function, the exact name of this function (i.e., case-sensitive) is used.
- When referring to a variable, it is done with regards to the scope of the variable (e.g., call local variables locally).
- Local variables are declared and can be used within the same function.
- Global variables are declared outside functions and can be used anywhere within a script.
Common errors and Best Practices
As you will start your journey through JavaScript coding, you may sometimes find it difficult to interpret the errors produced by the browser. However, after some practice, you will manage to recognize them, to understand (and also avoid) them, and to fix them accordingly. The next list identifies the errors that my students often come across when they start coding in JavaScript.
When your script contains an error, the browser usually provides you with enough information to check where it has occurred, so that you can fix it. While many bugs are relatively obvious to spot, some others are trickier to find. In the following, I have listed some of the most common errors that you will come across as you start with JavaScript.
The trick is to recognize the error message so that you can understand what the browser is trying to tell you. Again, this is part of the learning process, and you WILL make these mistakes; however, the more you see these errors, and the more you will learn to understand them (and avoid them too :-)).
Again, the browser is trying to help you by communicating, to the best that it can, where the issue is. By understanding the error messages, we can get to fix them easily.
The browser usually provides the following information when an error occurs:
- The name of the script where the error was found.
- The row and column where the error was found.
- A description of the error.
As we will see in the next sections, error messages are displayed in the console window. The console window is a usually provided in most modern browsers and makes it possible to locate errors. In most browsers, the console window can be accessed using the F12 key.
On the following figure, you can see that the browser indicates that an error has occurred. The message says “Assets/Scripts/myFirstScript.js (23,34) BCE0085: Unknown identifier: ‘localVariable’”. So the browser is telling us that an error has occurred in the script called myFirstScript, at the line 23, and around the 34th character (i.e., column) on this line. In this message, it is telling us that it can’t recognize the variable localVariable.
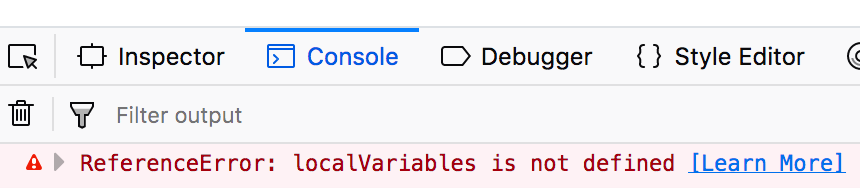
Figure 1‑12: Interpreting errors
To ensure that your code is easy to understand and that it does not generate countless headaches when trying to locate errors, there are a few good practices that you can start applying as you start coding; these should save you some time along the line by avoiding obvious errors.
Variable naming
- Use meaningful names that you can understand, especially after leaving your code for two weeks.
var myName = "Patrick";//GOOD var b = "Patrick";//NOT SO GOOD
- Capitalize the initial of all words in the name, except for the first word.
var testIfTheNameIsCorrect = true;// GOOD var testifthenameiscorrect = true; // NOT SO GOOD
Functions
- Use unique (i.e., different) names (for global and local variables).
- Check that all opening brackets have a corresponding closing bracket.
- Indent your code.
- Comment your code as much as possible to explain how it works.